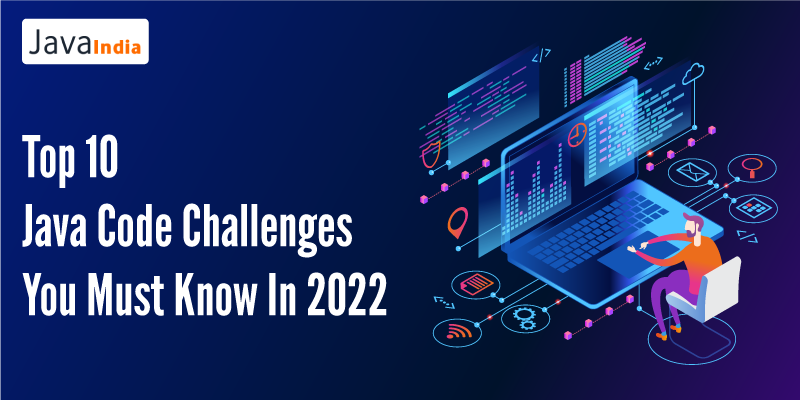
Top 10 Java Code Challenges You Must Know in 2022
Java code challenges are important parts of the Java certification process. And! They’re also fun ways to flex your coding skills, whether you’re just learning Java or you’ve been coding for years already.
But the problem with these Java code challenges is that they can be difficult to solve! In fact, some of them are so tricky that students who are new to coding often get discouraged from continuing their pursuit of certification, thinking it’s not possible.
That’s why it’s important to learn how to conquer these 10 advanced Java code challenges!
- String array to String
Converting an array of strings to a single string can be accomplished in a few different ways. The most common way is to use the String.join() method, which takes an array of strings and a delimiter as arguments and returns a single string.
However, this only works for arrays with a size greater than or equal to 2. If you have an array with only one element, you can use the Array.toString() method, which will return a string representation of the array.
Otherwise, you may want to try implementing recursion by starting from the end of the array and working your way back up. Another option is to use loops by iterating through each element in the array until it’s empty and then adding that item to a new string that represents all elements combined using concatenation (the + operator).
- String array length
You might be surprised to learn that the length of a string array can be calculated in a number of ways. The most common way is to use the String.length() method, which returns the number of characters in the string.
However, this only works for arrays of strings that are all the same length. What if you have an array of mixed lengths? There’s no reliable way to calculate the length of an array like this with one single command.
A better option would be to iterate through the array and count up how many items you’ve visited, then return that total at the end.
- Create Java Singleton Class
Undoubtedly, it is one of the greatest Java code challenges. A Singleton class is a code segment class that can have only one instance at a time. It is useful for creating global variables or for managing access to resources that need to be shared across the application. In order to create a Singleton class, you need to follow these steps:
1) Make the constructor private so that no other class can instantiate it.
2) Create a static method that returns an instance of the Singleton class.
If you are a company, you can easily hire Java developers in India that establish such a class.
- Code a program that returns an MD5 hash
The MD5 Message-Digest in Java is a cryptographic hash function. It takes an input of any length and returns a 128-bit string. A cryptographic hash function is a mathematical algorithm that takes an input of any length and converts it to a seemingly random output. MD5 hashes are used for the verification of data integrity, message authentication codes, and digital signatures.
- Writing three lambda functions
This Java code challenge will test your ability to write lambdas (i.e., anonymous functions). Lambdas can be thought of as mini-functions that are able to take in a few parameters and return a value.
In this challenge, you’ll be writing three of them:
One that returns true if the number passed is odd and false for even numbers;
one for determining whether or not the number is prime;
one for determining whether or not the parameter itself is palindromic (i.e., repeats the same letter from left to right).
- Write a phone number word decoder
Write a program in Java that will input phone numbers with letters and convert them to phone numbers with only digits.
Note that the user shouldn’t just replace all letters for the digits they represent but also properly format the number using parentheses and dashes. If an invalid number is given, then an error message should be thrown.
- Create a method to determine consecutive numbers
Create a function that accepts an array of integers as a parameter. If the contents of the input array are arranged consecutively (ie. there are no repeated integers) then the function should return true; otherwise, it will return false for invalid input.
The input array may be of any length. Since every Java software development company train developers on these challenges, you can learn to break through easily.
- Recognize the hex code in a string
In this challenge, you’ll create a method in Java that accepts both a string to be searched and a string that’s been encoded in hexadecimal (hex) and embedded in the first string as parameters. The function should find the first parameter and return the index of the second parameter.
- Write a Pig Latin translator
Pig Latin is a language or language game. Here are the steps for any word:
1) Move the first consonant or consonant cluster to the end of the word.
2) Adding ay in the word’s end
But! Coder can do it with some extra effort.
- Reverse only the letters in a string
Code a function in Java that accepts a string as a parameter. This function should provide a string that reverses the letters in the string sent as a parameter. But! It must keep all the numbers in order.
Wrapping Up
While these challenges may seem daunting at first, remember that practice makes perfect. The more you code, the better you’ll become at solving problems quickly and efficiently. So don’t get discouraged—keep at it, and eventually, you’ll be able to tackle anything that comes your way.
For an enterprise, it can be hard to implement all these coding aspects with an inexperienced developer. So, it is essential they hire Java developers in India with adequate expertise.
FAQs:
Is Java coding easy?
Java is straightforward to study. Java turned into designed to be clean to apply and is consequently clean to write, compile, debug, and study than different programming languages. Java is object-oriented. This permits you to create modular applications and reusable code.
Is Java or Python better?
Although Java is faster, Python is more versatile, less complicated to read, and has a less complicated syntax. According to Stack Overflow, this widespread use, interpreted language is the fourth maximum famous coding language.
Why is Java so tough?
Learning Java is simplest tough if you have no technical history otherwise you do not take the right steps to study the language. Java syntax is a statically typed gadget language that has huge functions and frameworks and may be included in numerous platforms.