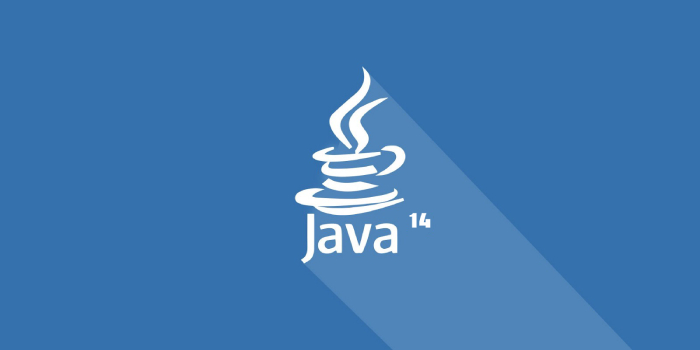
Java 14 Arrived: What’s New for Web Application Development?
Java 14 and its open-source Java Development Kit 14 has been released for developers, the most popular coding language for application development in the world. With version 14 significant numbers of Java Enhancement Proposals (JEPs) have been introduced for usage.
JAVA 14 addresses several remarkable features ranging from the Java language support to the latest APIs. So what is in store for the Java developers who write and maintain code for Java development services on a daily basis? The entire feature list comprises of:
- Switch Expressions
In Java 14 release, switch expressions become permanent. In earlier releases of Java, switch expressions were offered as “preview” feature. The new switch expressions ensure fewer errors within the code due to the absence of fall-through behaviour, exhaustiveness, and ease of writing. This is all possible because of the expression and compound form. A switch expression can leverage the arrow syntax, as shown in the example:
var log = switch (event) {
case PLAY -> "User has triggered the play button";
case STOP, PAUSE -> "User needs a break";
default -> {
String message = event.toString();
LocalDateTime now = LocalDateTime.now();
yield "Unknown event " + message +
" logged on " + now;
}
};
- Text Blocks
Java 13 was released with text blocks as a preview feature. This feature makes it simple to work with multiline string literals. This feature is released again with the Java 14 and includes a couple of tweaks. It is quite common to write code with different string concatenations and escape sequences to provide proper multiline text formatting. This is an important feature used by Java web Development Company during any development work. The code below shows an example for HTML formatting:
String html = "<HTML>" +
"\n\t" + "<BODY>" +
"\n\t\t" + "<H1>\"Java 14 is here!\"</H1>" +
"\n\t" + "</BODY>" +
"\n" + "</HTML>";
You can simplify this process by writing more elegant code using text blocks like:
String html = """
<HTML>
<BODY>
<H1>"Java 14 is here!"</H1>
</BODY>
</HTML>""";
- Records
Record is another preview language feature in Java 14. Like other ideas, this feature helps to reduce verbosity in Java and helping developers to write more concise code. Records focus on certain domain classes which store data in fields and do not declare any custom behaviours.
Here’s a fully generated implementation for the BankTransaction class:
public class BankTransaction {
private final LocalDate date;
private final double amount;
private final String description;
public BankTransaction(final LocalDate date,
final double amount,
final String description) {
this.date = date;
this.amount = amount;
this.description = description;
}
public LocalDate date() {
return date;
}
public double amount() {
return amount;
}
public String description() {
return description;
}
@Override
public String toString() {
return "BankTransaction{" +
"date=" + date +
", amount=" + amount +
", description='" + description + '\'' +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
BankTransaction that = (BankTransaction) o;
return Double.compare(that.amount, amount) == 0 &&
date.equals(that.date) &&
description.equals(that.description);
}
@Override
public int hashCode() {
return Objects.hash(date, amount, description);
}
}
This provides a way to remove the verbosity and release a class that only aggregates data together with the implementations of the equals, hashCode, and toString methods.
- NullPointer Exceptions
Some developers consider throwing NullPointerExceptions like new “Hello world” in Java because it is impossible to escape from them. Jokes aside, they cause problems because they often appear in application logs when code is running in a production environment, which can make the debugging hard because the original code is not readily present. For example, consider the code below:
var name = user.getLocation().getCity().getName();
Before Java 14, the following error is displayed:
Exception in thread "main" java.lang.NullPointerException
at NullPointerExample.main(NullPointerExample.java:5)
Now, with release of Java 14, there’s a new JVM feature that can receive more-informative diagnostics:
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "Location.getCity()" because the return value of "User.getLocation()" is null
at NullPointerExample.main(NullPointerExample.java:5)
These are some of the important features introduced with Java 14 and to build a web application having these features, you can hire Java developers from a reputed organization having appropriate resources for the development work.
Frequently Asked Questions
- Is Java 14 a LTS?
Java 14 was released in March 2020. It is not an LTS release and will be outdated at the release of Java 15 in September 2020.
- What does Java LTS mean?
A Java LTS (long-term support) release is a version of Java that will remain the industry standard for several years. For example, Java 8 was released in 2014, it will continue to receive updates until 2020, and extended support will end by 2025.
- What is the purpose of JDK?
The Java Development Kit (JDK) is a software development environment used for developing Java applications and applets. It includes the Java Runtime Environment, an interpreter/loader, a compiler (javac), an archiver (jar), a documentation generator and other tools needed in Java development.
Wrapping Up:
In Java 14, there are some remarkable features and updates that help developers in web application development. For example, Java 14 introduces an instance of pattern matching, which helps to reduce explicit casts. Records are available which declare classes that are used solely to aggregate data. Text blocks, a feature that helps to work with multiline string values. One other significant change is the event streaming in the JDK Flight Recorder. All these features make it the perfect choice for Java J2ee development and other development activities.